Texting sweet nothings with Lambda and Twilio
For Avital’s birthday, I wrote an app that texts her sweet messages several times per week – she loves it! I built it with Amazon Lambda and Twilio and it was pretty quick; I’ll walk you through it in this post.
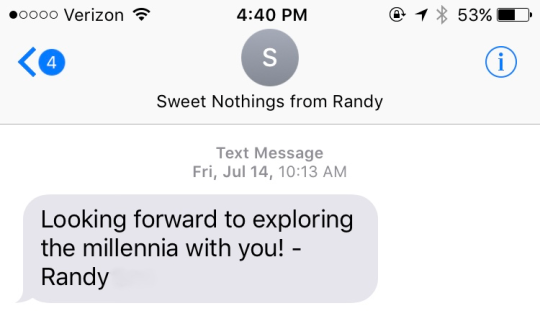
Tech Stack Overview
The app has is built on two services, Amazon Lambda and Twilio.
Lambda is part of Amazon Web Services (AWS) and allows you to run a short snippet of code on demand – this is much better than the alternative of maintaining a server just to run an app a few times per day. You can sign up for a free AWS account which should more than cover your Lambda usage.
Twilio is platform that allows you to easily integrate your code with SMS, voice calls, and other telecommunications infrastructure. For this app, we’ll only use their texting. You can try it for free but you’ll want to upgrade to their paid version once you’re ready to share it with your partner. It’s cheap though: $0.0075 per text message plus $1 per month for the phone number.
Setting up Lambda and Twilio
I followed this guide to get the initial Lambda and Twilio integration working. The guide is well written and has photos to guide you through the process. I didn’t have any issues getting an initial text sent to my phone.
Once I had their integration working, I customized my code to send out sweet messages to my fiancée. You can see my updated code in this GitHub Gist. Feel free to copy and past it into Lambda and overwrite the Twilio code.
The key functionality is in 24-36, where you can write a list of messages and a list of sign-offs (signatures). Line 36 randomly assembles the body of the text from the two lists. Having a great list of messages is key to having your partner like the app!
# add your own sweet nothings to this array
message_list = [
"I love you!",
"I am so lucky to have you in my life!"
]
# put your own name, sign-offs, pet names in this list
sign_off_list = [
"Randy",
"Fiance"
]
body = random.choice(message_list) + " -" + random.choice(sign_off_list)
Another significant change is the addition of environmental variables MY_NUMBER and PARTNERS_NUMBER. These are custom environmental variables that you’ll add on the Lambda page (below the code) just like you added the Twilio Auth Token and account ID. MY_NUMBER is the custom phone number that you have from Twilio and PARTNERS_NUMBER is your partner’s real personal phone number (or your personal number while you test the app). The format should be +15554442233 – don’t forget the `+1` and don’t use any quotation marks.
The final addition of note is the variable send_this_time which is randomly assigned to be true or false. I added this because I wanted some randomness around how often the script would send the text. You can play with the number to change the probability of the message sending. When you’re testing the code you can just set the variable to True so that it sends every time.
The final bit of setup is to instruct Lambda how often to trigger the script; here are the steps:
- Go to the Triggers Tab and click Add trigger
- Select CloudWatch Events
- Select Add a New Rule and make up a name and description for your script
- Add a Schedule Expression - this is the key step and there are further details below
- Submit the Trigger
Here’s a screenshot of the instructions filled out:
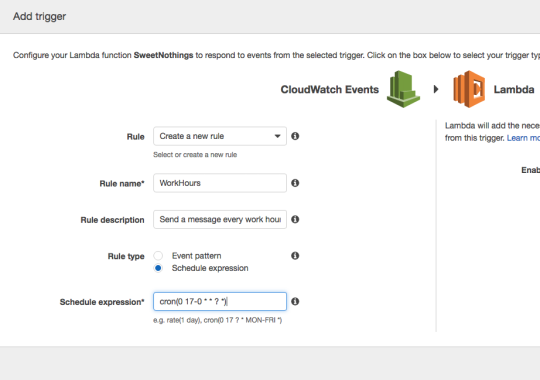
Schedule expressions can be rates (e.g. once per day) or for Crons (time based instructions. I used the expression cron(0 17-0 * * ? *) which runs the script once per hour during the work day (pacific time). You can learn more about scheduling events with Lambda on this Amazon page. It may take some trial and error to get the time zone right. Be aware, this controls how often the script runs but if you are using the send_this_now randomization, it won’t send every time. With the send_this_now frequency at 0.075, it sends a message - on average - about four times per week.
With the Cron Trigger set, Lambda will start executing the script and your partner should start getting texts. A few additional notes:
- Test with your own phone number to make sure everything works smoothly
- You need to add verify your partner’s number with Twilio
- Save the number in your partner’s contacts with something nice – I renamed it to Sweet Nothings from Randy
- Keep adding content to the message array so there’s always something new being sent
Good luck and let me know if you have any issues!